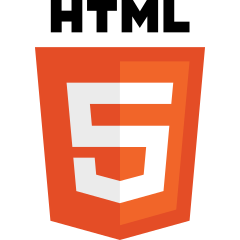
Structuring a Website
WELCOME!
Interested in JavaScript?
You came to the right place!
Check it out!
Structuring a Website
Adding Style to Structure
Engineering the Fun
JavaScript Basics
If you master these fundamentals, you have a head start on coding in other languages too!
var array = [ "hello", "world", 1, true, "Team Awesome is the best!" ]
array[1]
contain?
Show Answer
JavaScript Arrays
Arrays (also known as lists) are used to store data!
JavaScript Functions
It's good practice to have a function for each task rather than multiple tasks in one function!
Follow along with this simple 3 step tutorial!
In order to use Dog API we need to understand what is going on first.
Purpose
Requirements
Grab the image element, and button(s) from the HTML
**Notice how I also created a variable called url which holds part of the dog api URL**
Create an event listener for the button(s)
**Notice how we created a loop since we grabbed ALL buttons of a class from Step 1**
Fetch the dog image and load it into our empty image element
**Notice how there is also other text added for the url**
**Notice that we are using data.message, this is what the Dog API has sent us back**
Show AnswerDog Image Generator